How to Create a Laravel Tagging System
August 10, 2022
In this post, I will show you how to create a Laravel Tagging System using the package from rtconner/laravel-tagging.
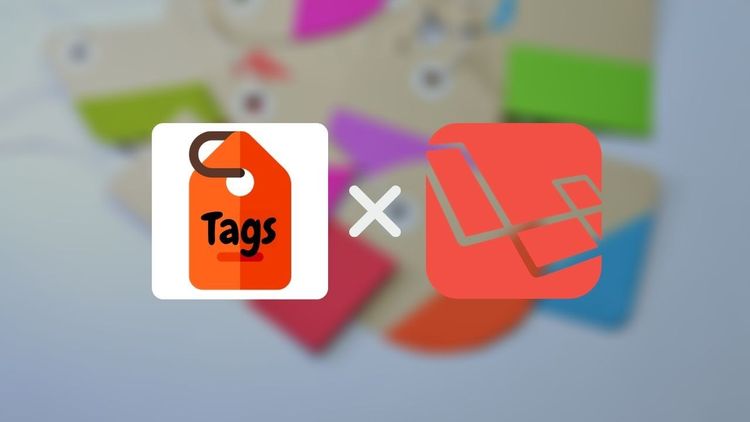
This blog is part of Packages that I use to build my Personal Blog with Laravel series
After previously creating a Subscription System, this is how we can create or manage an easy and free tag system with Laravel on this site before switch to Wordpress. This tagging system is needed when creating a site that contains articles or blogs. If you write a post for technology, you can create tags such as the name of the technology, type of technology, technology features, etc.
In this post, I will show you how to create a Laravel Tagging System using the package from rtconner/laravel-tagging. Here we assume we have a laravel project and then installed the laravel-tagging package.
Install Package Laravel Tagging System
In this step, we need to install the rtconner/laravel-tagging
package using composer
by running this command:
composer require rtconner / laravel-tagging
Publish and run the migration
The package will autodiscover when updating composer. Then publish tagging.php
and run the database migration with this command:
php artisan vendor:publish --provider=""Conner\Tagging\Providers\TaggingServiceProvider""
php artisan migrate
Setup Models
Then prepare the models that will be used, assume models Post.php
, by adding this line use \Conner\Tagging\Taggable;
into the class.
{
use \Conner\Tagging\Taggable;
protected $fillable = ['title','tags','description'];
}
Controller Setup
In the controller that will be used, assume PostController.php
, in the store data section add a line to enter the tag data into the database. Adjust the code below:
{
public function store(Request $request)
{
$this->validate($request, [
'title' => 'required',
'description' => 'required',
'tags' => 'required',
]);
$input = $request->all();
$tags = explode("", "", $input['tags']); // pecahkan string ke array tags
$post = Post::create($input); // data post ke db
$post->tag($tags); // data array tags ke db
return back()->with('success','Post created successfully.');
}
}
Setup View
The layout can be customized, or you can use Bootstrap, JQuery and Bootstrap Tags Input. To use Bootstrap Tags Input just add data-role=""tagsinput""
to the input field which will automatically convert it to the tag input field. Customize the example below:
<form method=""POST"" action=""{{ route('posts.store') }}"">
...
<div class=""form-group"">
<label>Tags : <span class=""text-danger"">*</span></label>
<br>
<input type=""text"" data-role=""tagsinput"" name=""tags"" class=""form-control tags"">
<br>
@if ($errors->has('tags'))
<span class=""text-danger"">{{ $errors->first('tags') }}</span>
@endif
</div>
...
</form>
To display the tag that has been entered together with the post, you can run the following command:
@foreach($posts as $post)
...
<div class=""post-tags mb-4"">
<strong>Tags : </strong>
@foreach($post->tags as $tag)
<span class=""badge badge-info"">{{$tag->name}}</span>
@endforeach
</div>
...
@endforeach
That's it! The tag system using laravel is ready to use. For more information or other usage examples of this package, see the github rtconner/laravel-tagging page.